OpenGL Programming/Migrating from 1.x to 2.x
OpenGL ES 2.0 has most of OpenGL 1.x just not available, and OpenGL 3.x and OpenGL 4.x deprecate it (the features are still available in the "compatibility profile", but you can expect the features to disappear soon enough).
However, little is said about how these features are replaced in the new versions. Here's how:
Matrix stack
[edit | edit source]Deprecated functions: glIdentity, glRotate, glOrtho, gluOrtho...
Matrix maths can be done:
- in the shaders, using build-in operators
- in the C source code using additional libraries such as:
GLM provides glm::ortho to replace glOrtho and gluOrtho for orthographic perspectives (and 2D programming).
Examples:
- Tutorial 05: cube rotation
Vertex and texture
[edit | edit source]Deprecated functions: glVertex, glTexCoord...
These data are now sent in batch mode, as C arrays. You can use:
- directly a C array : data is resent a each frame
- glBufferData : send the C data once to the graphic card, then refer to the buffer
You use glVertexAttribPointer, once for each vertex attribute (position, color, texture coordinate...), passing it the C array or OpenGL buffer.
Examples:
- Tutorial 01: introduction (C arrays, no buffer)
- Tutorial 04: cube (elements)
Then you use glDrawArrays or glDrawElements to start drawing.
Normals
[edit | edit source]Deprecated functions: glNormal, glNormalPointer
Normals need to be passed to the shaders (namely for shading computations), like vector coordinates (glVertexAttribPointer). To compute the normal of 3 vertices a, b and c in counter-clockwise order, GLM provides:
glm::normalize(glm::cross(b - a, c - a)); glm::fastNormalize(glm::cross(b - a, c - a)); // faster but less accurate
Examples:
- Tutorial 08: load .obj, computing normals on the fly
Evaluators - Bezier curves and surfaces
[edit | edit source]Deprecated functions: glMap1, glMap2, glEvalCoord, glEvalPoint...
- GLU ES - http://code.google.com/p/glues/ - this is for OpenGL 1.x, so it may not work in a 2.x environment, but provides replacement code
- Manual code (not that hard)
Examples:
- Tutorial 07: teapot (Bézier surfaces)
Lighting
[edit | edit source]Deprecated functions: glLight (glLightfv3, etc.), glShadeModel...
The bad news is that you have to reimplement everything in the shaders. The good news is that it's not so hard, plus you have copy/pasteable code in the examples below :)
You also need to make the light parameters available to your shaders, and it's best to store them as a shader struct
.
Examples:
- Diffuse Reflection
- Other parts of the lighting tutorials
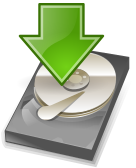