QBasic/Appendix
Commands
[edit | edit source]ABS()
[edit | edit source]N = ABS(expression returning a numerical value)
Returns the 'absolute' value of the expression, turning a negative to a positive (e.g. -4 to 4)
PRINT ABS(54.345) 'This will print the value ABS now as it is (54.345)
PRINT ABS(-43) 'This will print the value as (43)
.
ACCESS
[edit | edit source]OPEN "file.txt" FOR APPEND ACCESS WRITE
This sets the access of a file that has been declared into the program. There are three settings that the programmer can set. These are:
READ - Sets up the file to be read only, no writing. WRITE - Writes only to the file. Cannot be read. READ WRITE - Sets the file to both of the settings above.
FOR APPEND opens the file as a text file and sets the file pointer to the end of the file, thereby appending new output to the file. If omitted, FOR RANDOM is the default, which would open the file as a sequence of fixed-length records and set the file pointer to the start of the file. New data would overwrite existing data in the file without warning.
ASC("C")
[edit | edit source]PRINT ASC("t") 'Will print 116
Prints the ASCII code number of the character found within the brackets. If the programmer put in a string into brackets, only the first character of the string will be shown.
ATN()
[edit | edit source]ATN(expression)
Part of the inbuilt trigonometry functions. An expression that evaluates to a numeric vale is converted to it's Arc-Tangent.
angle = ATN( B ) angle2 = ATN( 23 / 34 )
BEEP
[edit | edit source]BEEP
The BIOS on the motherboard is instructed to emit a "Beep" sound from the PC 'speaker'. See also SOUND and PLAY.
An older, outdated alternative is to use : PRINT CHR$(07)
This was replaced later by the BEEP command.
BLOAD
[edit | edit source]BLOAD file_path$, memory_offset%
Loads a file saved with BSAVE into memory.
- file_path$ is the file location
- memory_offset is an integer specifying the offset in memory to load the file to
The starting memory address is determined by the offset and the most recent call to the DEF SEG statement
BSAVE
[edit | edit source]BSAVE file_path$, memory_offset%, length&
Saves the contents of an area in memory to a file that can be loaded with BLOAD
- file_path$ is the file location
- memory_offset is an integer specifying the offset in memory to save
- length the number of bytes to copy
The starting memory address is determined by the offset and the most recent call to the DEF SEG statement
CALL ABSOLUTE
[edit | edit source]CALL ABSOLUTE([argument%, ...,] address%)
Pushes the provided arguments, which must be INTEGER, from left to right on the stack and then does a far call to the assembly language routine located at address. The code segment to use is set using DEF SEG. Normally QBasic will push the address of arguments, but if an argument is preceded by BYVAL the value of the argument will be pushed.
Note that because QBasic pushes the arguments from left to right, if you provide three arguments for example the stack will look like this:
SS:SP Return IP +0002 Return CS +0004 Argument 3 +0006 Argument 2 +0008 Argument 1
Example:
'POP CX 'POP DX 'POP BX 'POP AX 'MOV [BX], AX 'PUSH DX 'PUSH CX 'RETF A$ = "YZ[Xë•RQ╦" 'Codepage: 437 I% = 42: J% = 0 IF VARSEG(A$) <> VARSEG(J%) THEN STOP 'Both A$ and J% are stored in DGROUP. DEF SEG = VARSEG(A$) CALL ABSOLUTE(BYVAL I%, J%, PEEK(VARPTR(A$) + 3) * 256& OR PEEK(VARPTR(A$) + 2)) PRINT J%
QBasic doesn't set BP to the stack frame of your machine language routine, but leaves it pointing to the stack frame of the calling QBasic procedure, which looks like this:
-???? Variable 3 -???? Variable 2 -???? Variable 1 -???? Return value (only if the procedure is a FUNCTION, absent if a SUB) -0002 BP of calling procedure SS:BP BP of calling procedure (yes, again) +0002 Six bytes referring back to the calling procedure to use when executing END/EXIT SUB/FUNCTION +0008 Argument 3 +000A Argument 2 +000C Argument 1
The offsets indicated with -/+???? will depend on the sizes and presence of variables, return value and arguments. For example, an INTEGER variable will take two bytes, but a LONG variable four. As one might expect considering how QBasic passes arguments, variables are stored in reverse order of declaration. Contrary to when calling a machine language routine, the arguments here will always be addresses. For arguments passed by value, space is allocated on the stack and the address of that space is passed to the procedure.
CASE
[edit | edit source]SELECT CASE expression CASE test1[, test2, ...]: statements [CASE test4[, test5, ...]: statements] [CASE ELSE: statements] END SELECT
Executes the statements after the first CASE statement where a test matches expression. The tests can be of the following forms:
expression expression1 TO expression2 IS {<|<=|=|<>|>=|>} expression
This is an example of a program with no CASE commands that assigns different paths to values:
PRINT "1. Print 'path'" PRINT "2. Print 'hello'" PRINT "3. Quit" INPUT "Enter a choice: "; a$ IF a$ = "1" THEN PRINT "path": RUN IF a$ = "2" THEN PRINT "hello": RUN IF a$ <> "3" THEN PRINT "That is not a valid choice.": RUN
This is what a program looks like with the CASE command:
PRINT "1. Print 'path'" PRINT "2. Print 'hello'" PRINT "3. Quit" INPUT "Enter a choice: "; a$ SELECT CASE a$ CASE "1": PRINT "path": RUN CASE "2": PRINT "hello": RUN CASE IS <> "3": PRINT "That is not a valid choice.": RUN END SELECT
CHAIN
[edit | edit source]CHAIN filename
This chains execution to another QBasic program. Values may be passed to the other program by using the COMMON statement before the CHAIN statement. Note that execution doesn't return to the first program unless the second program uses CHAIN to transfer execution back to the first.
CHDIR
[edit | edit source]CHDIR directoryname
This is used for setting the working directory, also known as the current directory. The directory name is declared exactly like in DOS and long file names aren't supported. For example:
CHDIR "C:\DOS"
Note that this doesn't change the current drive and every drive has its own working directory. You can set the current drive like this:
SHELL "C:"
CHR$()
[edit | edit source]This returns the string character symbol of an ASCII code value.
name$ = CHR$([ascii character code])
Often used to 'load' characters into string variables when that character cannot be typed (e.g. the Esc key or the F{n} (Function Keys) or characters that would be 'recognised' and acted upon by the QBASIC interpreter. The following four characters cannot occur in a QBasic string literal:
- 0 Null: all characters up to the end of the line will get deleted, including this one.
- 10 Line Feed: signals the end of the line.
- 13 Carriage Return: this character will get deleted.
- 34 Quotation Mark: signals the end of the string literal.
Here is a list of some character codes :-
07 Beep (same as BEEP) 08 Backspace 09 Tab 27 Esc 72 Up Arrow 75 Left Arrow 77 Right Arrow 80 Down Arrow
CINT()
[edit | edit source]This rounds the contents of the brackets to the nearest integer.
PRINT CINT(4573.73994596)
4574
CIRCLE
[edit | edit source]CIRCLE ([X Coordinate], [Y Coordinate]), [Radius], [Colour],[Start],[End],[Aspect]
Lets the programmer display a circle. Like all graphics commands, it must be used with the SCREEN command.
CLEAR
[edit | edit source]CLEAR
Resets all variables, strings, arrays and closes all files. The reset command on QBasic.
CLOSE
[edit | edit source]CLOSE
Closes all open files
CLOSE #2
Closes only the file opened as data stream 2. Other files remain open
CLS
[edit | edit source]CLS
Clears the active screen. Erases all text, graphics, resets the cursor to the upper left (1,1), and also applies the current background color (this has to be set using the COLOR command) to the whole screen.
COLOR
[edit | edit source]COLOR [Text Colour], [Background Colour]
This lets you change the colour of the text and background used when next 'printing' to the current output window. It can be done like this:
COLOR 14, 01 PRINT "Yellow on Blue"
You have a choice of sixteen colours:
00: Black 08: Dark Grey 01: Dark Blue 09: Light Blue 02: Dark Green 10: Light Green 03: Dark Cyan 11: Light Cyan 04: Dark Red 12: Light Red 05: Dark Purple 13: Magenta 06: Orange Brown 14: Yellow 07: Grey 15: White
These values are the numbers that you put in the COLOR command.
Note Only screen modes 0, 7, 8, 9, 10 support a background color. To 're-paint' the whole screen in a background colour, use the CLS command.
COMMON
[edit | edit source]Declares a variable as 'global', which allows its value to be accessed across multiple QBasic programs / scripts (see also the CHAIN command)
COMMON SHARED [variablename]
Each program that declares 'variablename' as COMMON will share the same value.
NOTE. All COMMON statements must appear at the start of the program (i.e. before any executable statements).
CONST
[edit | edit source]Fixes a variable so it can not be changed within the program.
CONST (name) {AS (type = INTEGER / STRING)} = (expression or value)
For example :-
CONST PI = 3.14159265
Assigns the value 3.14159265 to PI.
CONST PI2 = 2 * PI
PI must be assigned a value before it is used to calculate PI2. Typically all CONST are declared at the beginning of a program.
DATA
[edit | edit source]DATA [constant]
Use in conjunction with the READ and RESTORE command. Mostly used in programs dealing with graphics, this command lets QBasic read a large number of constants. The READ command accesses the data while the RESTORE command "refreshes" the data, allowing it to be used again.
DATE$
[edit | edit source]A system variable that always contains the current date as a string in mm-dd-yyyy format. Use it like this:
a$ = DATE$
DEF SEG
[edit | edit source]Sets the current segment address.
DEG SEG [=address]
- address is a segment address that can contain a value of 0 through 65535.
If address is omitted, DEF SEG resets the current segment address to the default data segment. DEF SEG is used by BLOAD, BSAVE, CALL ABSOLUTE, PEEK, and POKE
DEST(Only QB64!)
[edit | edit source]_DEST sets the current write-to page or image. _DEST image_handle sends the destination image to an image of handle stored in long variable image_handle. _DESt 0 sends the destination image to the current screen being used.
DIM
[edit | edit source]This is used to declare an array (early versions of QBasic required all variables to be defined, not just arrays greater than 10)
DIM [Array Name] ([count],[count], ..)
The Array name can be of any type (Integer, Double, String etc). If not declared, single precision floating point is assumed. Strings can be 'declared' using $ sign (Integers with the '%' sign). The QBASIC interpreter tolerates numeric arrays of up to 10 count without these needing to be declared.
NOTE Early versions of QBasic did not explicitly set the contents of an array to zero (see CLEAR command)
DIM table%(100,2)
Create an integer array called table% containing 100x2 = 200 entries
DIM form$(5)
Create a string array called form$ containing 5 strings
DIM quotient(20) AS DOUBLE
Create an array called quotient that contains 20 double precision numbers
DO .. LOOP
[edit | edit source]DO [program] LOOP UNTIL [test condition becomes TRUE]
Used to create a loop in the program. The [condition] is tested only after the [program] code is executed for the first time (see also WHILE). For example:
num$ = 1 sum$ = 0 DO sum$ = 2 * num$ PRINT sum$ num$ = num$ + 1 LOOP UNTIL num$ = 13
This does not work But the following does
num = 1 sum = 0 DO sum = 2 * num PRINT sum num = num + 1 LOOP UNTIL num = 13
DRAW
[edit | edit source]DRAW "[string expression]"
Used to draw a straight line from the current 'cursor' position in the current colour. DRAW defines the direction (up, down etc.) and the length of the line (in pixels). For example:-
SCREEN 7 PSET (50, 50), 4 DRAW "u50 r50 d50 l50"
The letter in front of each number is the direction:
U = Up E = Upper-right D = Down F = Lower-right L = Left G = Lower-left R = Right H = Upper-left
The drawing 'cursor' is left at the position where the line ends. u50 draws from 50,50 upwards ending at 50,0 r50 draws from 50,0 to the right, ending at 100,0 d50 draws from 100,0 downwards, ending at 100,50 l50 draws from 100,50 to the left, ending at 50,50
The example shown will thus draw a red 'wire frame' square.
See also LINE and CIRCLE commands.
Note: The diagonal from 0,0 to 100,100 will be 100 * root(2) pixels long (i.e. 141)
END
[edit | edit source]END
Signifies the end of the program. When QBasic sees this command it usually comes up with a statement saying: "Press Any Key to Continue".
END TYPE / END DEF / END SUB / END FUNCTION / END IF / END SELECT
[edit | edit source]END TYPE
ends a TYPE definition.END DEF
ends a DEF FN function definition.END SUB
ends a SUB procedure definition.END FUNCTION
ends a FUNCTION procedure definition.END IF
ends a multiline IF block.END SELECT
ends a SELECT CASE block.
ENVIRON
[edit | edit source]ENVIRON [string expression]
NOTE: If you are running QBasic on a Windows system, you will not be able to use this command.
This command helps you set an environment variable for the duration of the session. On exit from the QBasic.exe interpreter, the variables revert to their original values.
EOF()
[edit | edit source]This checks if there are still more data values to be read from the file specified in (). EOF() returns a boolean / binary value, a one or zero. 0 if the end of file has not been reached, 1 if the last value in the file has been read (see also LINE INPUT)
OPEN File.txt FOR INPUT AS #1 DO INPUT #1, text$ PRINT text$ LOOP UNTIL EOF(1) END
Note that, since the INPUT is executed before UNTIL is reached, File.txt must contain at least one line of text - if the file is empty, you will receive an 'ERROR (62) Input past end of file'.
ERASE
[edit | edit source]ERASE [arrayname] [,]
Used to erase all dimensioned arrays.
ERROR
[edit | edit source]System variable holding a numeric value relating to the processing of the previous line of code. If the line completed without error, ERROR is set to 0. If the line failed, ERROR is set to one of the values shown below. Most commonly used to redirect program flow to error handling code as in :-
ON ERROR GOTO [line number / label]
If ERROR is non=zero, program flow jumps to the line number or label specified. If ERROR is zero, program flow continues with the next line below.
To manually test your program and check to see if the error handling routine runs OK, ERROR can be set manually :-
ERROR [number]
Set ERROR = number
The error numbers are as follows:
1 NEXT without FOR 39 CASE ELSE expected 2 Syntax Error 40 Variable required 3 RETURN without GOSUB 50 FIELD overflow 4 Out of DATA 51 Internal error 5 Illegal function call 52 Bad file name or number 6 Overflow 53 File not found 7 Out of memory 54 Bad file mode 8 Label not defined 55 File already open 9 Subscript out of range 56 FIELD statement active 10 Duplicate definition 57 Device I/O error 11 Division by zero 58 File already exists 12 Illegal in direct mode 59 Bad record length 13 Type mismatch 61 Disk full 14 Out of string space 62 Input past end of file 16 String formula too complex 63 Bad record number 17 Cannot continue 64 Bad file name 18 Function not defined 67 Too many files 19 Yes RESUME 68 Device unavailable 20 RESUME without error 69 Communication-buffer overflow 24 Device timeout 70 Permission denied 25 Device Fault 71 Disk not ready 26 FOR without NEXT 72 Disk-media error 27 Out of paper 73 Advanced feature unavailable 29 WHILE without WEND 74 Rename across disks 30 WEND without WHILE 75 Path/File access error 33 Duplicate label 76 Path not found 35 Subprogram not defined 37 Argument-count mismatch 38 Array not defined
Note that ERROR is set when execution fails, not when the code is 'read' - so, for example, a 'divide by 0' will be found before the result is assigned to a non-existent array variable or written to a non-existent file.
EXIT
[edit | edit source]Allows the immediate exit from a subroutine or a loop, without processing the rest of that subroutine or loop code
EXIT DEF
Exits from a DEF FN function.
EXIT DO
Exits from a DO loop, execution continues with the command directly after the LOOP command
EXIT FOR
Exits from a FOR loop, execution continues with the command directly after the NEXT command
EXIT FUNCTION
Exits a FUNCTION procedure, execution continues with the command directly after the function call
EXIT SUB
Exits a SUB procedure.
FOR .. NEXT
[edit | edit source]FOR [variable name] = [start value] TO [end value] {STEP n} [program code] NEXT [variable name]
The variable is set to the [start value], then program code is executed and at the Next statement the variable is incremented by 1 (or by the STEP value, if any is specified). The resulting value is compared to the [end value] and if not equal program flow returns to the line following the FOR statement.
For example:
FOR a = 200 TO 197 STEP-1 PRINT a NEXT a
200 199 198
Care must be taken when using STEP, since it is quite possible to step past the (end value) with the result that the FOR loop will run 'for ever' (i.e. until the user aborts the interpreter or an error occurs), for example :-
FOR a = 200 TO 197 STEP-2 PRINT a NEXT a
200 198 196 194 192 ... 0 -2 -4 ... -32768 ERROR overflow
GOSUB
[edit | edit source]GOSUB [subroutine line number / label]
Command processing jumps to the subroutine specified. When the RETURN command is encountered, processing returns to this point and continues with the line below the GOSUB.
IF
[edit | edit source]IF [variable or string] [operator] [variable or string] THEN [command] {ELSE [command]}
Compares variables or strings. For example, if you wanted to examine whether or not a user-entered password was the correct password, you might enter:
IF a$ = "password" THEN PRINT "Password Correct"
Where a$ is the user entered password. Some operators include:
"="- equal to
"<"- less than (only used when variable or string is a number value)
">"- greater than (only used when variable or string is a number value)
"<>"- does not equal
"<="- less than or equal to (only used when variable or string is a number value)
">="- greater than or equal to (only used when variable or string is a number value)
One can also preform actions to number values then compare them to other strings or variables using the if command, such as in the below examples:
IF a+5 = 15 THEN PRINT "Correct"
IF a*6 = b*8 THEN PRINT "Correct"
INCLUDE (QUICKbasic Only)
[edit | edit source]QUICKBasic supports the use of include files via the $INCLUDE directive:
(Note that the Qbasic interpreter does NOT support this command.)
'$INCLUDE: 'foobar.bi'
Note that the include directive is prefixed with an apostrophe, dollar, and that the name of the file for inclusion is enclosed in single quotation mark symbols.
INKEY$
[edit | edit source][variable] = INKEY$
This is used when you want a program to function with key input from the keyboard. Look at this example on how this works:
a$ = INKEY$ PRINT "Press Esc to Exit" END IF a$ = CHR$(27)
You can use this in conjunction with the CHR$ command or type the letter (e.g. A).
INPUT
[edit | edit source]INPUT [String Literal] [,or;] [Variable]
Displays the String Literal, if a semi colon follows the string literal, a question mark is displayed, and the users input until they hit return is entered into the variable. The variable can be a string or numeric. If a user attempts to enter a string for a numeric variable, the program will ask for the input again. The String Literal is option. If the string literal is used, a comma (,) or semicolon (;) is necessary.
INPUT #
[edit | edit source]INPUT #n [String Literal] [,or;] [Variable]
Reads a string / value from the specified file stream (see also LINE INPUT #)
INPUT #1, a$, b$, n, m
Reads 4 values from the file that is OPEN as #1. a$ is assigned all text until a ',' (comma) or end of line is reached, b$ the next segment of text, then two numeric values are interpreted and assigned to n and m.
Note that, within the file, numbers can be separated by 'anything' - so, if a number is not found (for 'n' or 'm') on the current 'line' of the file, the rest of the file will be searched until a number is found. Input is then left 'pointing' at the position in the file after the last number required to satisfy the input statement (see also 'seek #' command)
INSTR
[edit | edit source]INSTR (start%, Search$, Find$)
Returns the character position of the start of the first occurrence of Find$ within Search$, starting at character position 'start%' in Search$. If Find$ is not found, 0 is returned. start% is optional (default = 1, the first character of Search$)
Pos = INSTR ("abcdefghi", "de")
returns 4
LEFT$()
[edit | edit source]A$ = LEFT$(B$,N)
A$ is set to the N left most characters of B$.
A$ = LEFT$("Get the start only",6)
returns "Get th"
See also RIGHT$(), MID$().
LET
[edit | edit source]LET [variable] = [value]
Early versions of the QBasic.exe command interpreter required use of the 'LET' command to assign values to variables. Later versions did not.
LET N = 227 / 99 LET A$="a line of simple text"
is equliavent to :-
N = 227 / 99 A$="a line of simple text"
LINE
[edit | edit source]LINE ([X], [Y]) - ([X], [Y]), [Colour Number]
Used for drawing lines in QBasic. The first X and Y are used as coordinates for the beginning of the line and the second set are used for coordinating where the end of the line is. You must put a SCREEN command at the beginning of the program to make it work.
Note. When in SCREEN 13, the Colour Number == the Palette number
LINE INPUT #
[edit | edit source]LINE INPUT #1, a$
Reads a complete line as text characters from the file OPEN as stream #1 and places it in a$.
To find the 'end of line', the QBasic interpreter seaches for the 'Carriage Return' + 'Line Feed' (0x0D, 0x0A) characters. When reading text files created on UNIX/LINUX systems (where the 'Line feed' 0x0A is used on it's own to signify 'end of line'), LINE INPUT will not recognise the 'end of line' and will continue to input until the end of file is reached. For files exceeding 2k characters, the result is an "Out of String Space" Error as a$ 'overflows'. One solution is to use a text editor able to handle UNIX files to open and 'save as' before attempting to process the file using QBasic.
LOADIMAGE (QB64 Only)
[edit | edit source](NOTE! The commands in this section refer to a third-party program called "QB64". Neither QUICKbasic nor Qbasic support _LOADIMAGE, _NEWIMAGE, OR _PUTIMAGE commands. Both QUICKbasic and Qbasic have a "SCREEN" command, but it works diffently in those languages than in QB64.)
_LOADIMAGE("image.jpg")
Shows an image. Must be used with the commands SCREEN, _NEWIMAGE and _PUTIMAGE.
Example:
DIM rabbit AS LONG SCREEN _NEWIMAGE(800, 600, 32) rabbit = _LOADIMAGE("rabbit.jpg") _PUTIMAGE (100,100), rabbit
LOOP
[edit | edit source]DO [Program] LOOP UNTIL [condition]
Used to create a loop in the program. This command checks the condition after the loop has started. This is used in conjunction with the DO command.
LPRINT
[edit | edit source]LPRINT [statement or variable]
Prints out text to a printer. The LPRINT command expects a printer to be connected to the LPT1(PRN) port. If a printer is not connected to LPT1, QBasic displays a "Device fault" error message.
If your printer is connected to a COM port instead, use the MS-DOS MODE command to redirect printing from LPT1 to COMx (for example, to redirect to COM1, use the following command:
MODE LPT1=COM1
If you need to cancel the redirection when finished, use the following command:
MODE LPT1
MID$
[edit | edit source]a$=MID$(string$,start%[,length%]) MID$(string$,start%[,length%])=b$
In the first use, a$ is set to the substring taken from string$ strating with character start% taking Length% characters. If length% is omitted, the rest of the line (i.e. start% and all the characters to the right) are taken.
In the second use, length% characters of string$ are replaced by b$ starting at start%. If length% is omitted, the rest of the line is replaced (i.e. start% and all the characters to the right)
See also LEFT$ RIGHT$ LEN
MOD
[edit | edit source]a MOD b
Returns the remainder of an integer divide of a by b
For example, 10 MOD 3 returns 1
NEWIMAGE(Only QB64!)
[edit | edit source]_NEWIMAGE is used to set a long variable as the screen dimensions, or can be used with the SCREEN command (See later in Appendix) to directly set the screen details. It is very useful as you can enlarge the SCREEN mode '13' which has RGB color settings if you find the default size too small.
Syntax: _NEWIMAGE(width,length,screen_mode)
- width and length are long variables, while screen_mode is the screen mode format you wish to change.
Like,
_NEWIMAGE(1000,1000,24),256
where, 256 is the amount of colours
It is also used to prepare the window screen surface for the image you want to put (first load it using LOADIMAGE).
OPEN
[edit | edit source]OPEN "[(path)\8.3 file name.ext]" (FOR {INPUT/OUTPUT} AS #{n})
This opens a file. You have to give the DOS file name, for example:
OPEN "data.txt" FOR INPUT AS #1
Opens the existing file data.txt for reading as data stream #1. Since no path is specified, the file must be in the same folder as the QBasic.exe - if not, processing halts with a 'file not found' error
OPEN "C:\TEMP\RUN.LOG" FOR OUTPUT AS #2
Opens an empty file named RUN.LOG in the C:\TEMP folder for writing data stream #2. Any existing file of the same name is replaced.
PALETTE
[edit | edit source]PALETTE[palette number, required colour]
For VGA (SCREEN mode 13) only, sets the Palette entry to a new RGB color. The palette number must be in the range 1-256. The required colour is a LONG integer created from the sum of (required Blue * 65536) + (required Green * 256) + required Red.
RANDOMIZE
[edit | edit source]RANDOMIZE TIMER A = INT((RND * 100)) + 1
RANDOMIZE will set the seed for QBasic's random number generator. With QBasic, it's standard to simply use RANDOMIZE TIMER to ensure that the sequence remains the same for each run.
The example is a mathematical operation to get a random number from 1 to 100.
INT stands for Integer, RND for Random and "*" stands for the limit upto which the random number is to be chosen. The "+ 1" is just there to ensure that the number chose is from 1 to 100 and not 0 to 99.
Note: Subsequent calls of this function do not guarantee the same sequence of random numbers.
READ
[edit | edit source]READ AIM(I)
Used in conjunction with the DATA command, this command lets QBasic read data. This is mostly used when dealing with large quantities of data like bitmaps.
REM or '
[edit | edit source]REM {comments} ' {comments}
When the interpreter encounters REM or " ' " (a single quote) at the start of a line, the rest of the line is ignored
RETURN
[edit | edit source]RETURN
Signifies that it is the end of a subroutines
RND
[edit | edit source]RANDOMIZE TIMER A = INT((RND * 100)) + 1
RND will provide a random number between 0 and 1.
The example is a mathematical operation to get a random number from 1 to 100. RANDOMIZE TIMER will set the initial seed to a unique sequence. INT stands for Integer, RND for Random and "*" stands for the limit upto which the random number is to be chosen. The "+ 1" is just there to ensure that the number chose is from 1 to 100 and not 0 to 99.
Internally, the seed a 24-bit number, iterated in the following method: rnd_seed = (rnd_seed*16598013+12820163) MOD 2^24
PLAY
[edit | edit source]PLAY "[string expression]"
Used to play notes and a score in QBasic on the PC speaker. The tones are indicated by letters A through G. Accidentals are indicated with a "+" or "#" (for sharp) or "-" (for flat) immediately after the note letter. See this example:
PLAY "C C# C C#"
Whitespaces are ignored inside the string expression. There are also codes that set the duration, octave and tempo. They are all case-insensitive. PLAY executes the commands or notes the order in which they appear in the string. Any indicators that change the properties are effective for the notes following that indicator.
Ln Sets the duration (length) of the notes. The variable n does not indicate an actual duration amount but rather a note type; L1 - whole note, L2 - half note, L4 - quarter note, etc. (L8, L16, L32, L64, ...). By default, n = 4. For triplets and quintets, use L3, L6, L12, ... and L5, L10, L20, ... series respectively. The shorthand notation of length is also provided for a note. For example, "L4 CDE L8 FG L4 AB" can be shortened to "L4 CDE F8G8 AB". F and G play as eighth notes while others play as quarter notes. On Sets the current octave. Valid values for n are 0 through 6. An octave begins with C and ends with B. Remember that C- is equivalent to B. < > Changes the current octave respectively down or up one level. Nn Plays a specified note in the seven-octave range. Valid values are from 0 to 84. (0 is a pause.) Cannot use with sharp and flat. Cannot use with the shorthand notation neither. MN Stand for Music Normal. Note duration is 7/8ths of the length indicated by Ln. It is the default mode. ML Stand for Music Legato. Note duration is full length of that indicated by Ln. MS Stand for Music Staccato. Note duration is 3/4ths of the length indicated by Ln. Pn Causes a silence (pause) for the length of note indicated (same as Ln). Tn Sets the number of "L4"s per minute (tempo). Valid values are from 32 to 255. The default value is T120. . When placed after a note, it causes the duration of the note to be 3/2 of the set duration. This is how to get "dotted" notes. "L4 C#." would play C sharp as a dotted quarter note. It can be used for a pause as well. MB MF Stand for Music Background and Music Foreground. MB places a maximum of 32 notes in the music buffer and plays them while executing other statements. Works very well for games. MF switches the PLAY mode back to normal. Default is MF.
PRINT [Argument] [,or;] [Argument]...
Displays text to the screen. The Argument can be a string literal, a string variable, a numeric literal or a numeric variable. All arguments are optional.
PRINT #[n] [,or;] [Argument]...
Saves data to the file that is 'OPEN FOR OUTPUT AS #[n]' or we can use ? symbol for print command
PSET
[edit | edit source]PSET ([X coordinate],[Y coordinate]), [Pixel Colour]
This command displays pixels, either one at a time or a group of them at once. For the command to work, the program must have a SCREEN command in it.
SCREEN
[edit | edit source]SCREEN [Screen Mode Number]
This command is used for displaying graphics on the screen. There are ten main types of screen modes that can be used in QBasic depending on the resolution that you want. Here is a list of what screen modes you can choose from:
SCREEN 0: Textmode, cannot be used for graphics. This the screen mode that text based programs run on.
SCREEN 1: 320 x 200 Resolution. Four Colours
SCREEN 2: 640 x 200 Resolution. Two Colours (Black and White)
SCREEN 7: 320 x 200 Resolution. Sixteen Colours
SCREEN 8: 640 x 200 Resolution. Sixteen Colours
SCREEN 9: 640 x 350 Resolution. Sixteen Colours
SCREEN 10: 640 x 350 Resolution. Two Colours (Black and White)
SCREEN 11: 640 x 480 Resolution. Two Colours
SCREEN 12: 640 x 480 Resolution. Sixteen Colours
SCREEN 13: 320 x 200 Resolution. 256 Colours. (Recommended)
Note. In SCREEN 13 you have a colour Palette of 256 colours. The PALETTE is pre-set by Windows however you can change the RGB values using the PALETTE command.
SEEK
[edit | edit source]SEEK #[file number], 1
Repositions the 'input #' pointer to the beginning of the file.
SGN
[edit | edit source]SGN(expression yielding a numeric value)
Yields the 'sign' of a value, -1 if < 0, 0 if 0, 1 if > 0
SHELL
[edit | edit source]The 'SHELL' command is used in Qbasic to issue a command to Command Prompt/Windows Shell . The 'Shell' command is used along with a string that contains commands that would be understood by any of the above software. The string enclosed commands are much like that of MS-DOS
Example: SHELL can be used with a 'DIR' command to make a directory of files in a certain folder or path.
SLEEP
[edit | edit source]SLEEP [n]
Execution is suspended for n seconds
SOUND
[edit | edit source]SOUND [frequency], [duration]
Unlike the BEEP command, this produces a sound from the PC speakers that is of a variable frequency and duration. The frequency is measured in Hertz and has a range from 37 to 32767. Put in one of these numbers in the frequency section. The duration is clock ticks that is defaulted at 18.2 ticks per second.
STR$
[edit | edit source]Converts a numeric value into a text (string) character
A$ = STR$(expression yielding a numeric value)
The numeric value is converted into text characters and placed into A$. Use to convert numbers into a text string.
WARNINGS.
1) If the result is positive, a leading 'space' is added (STR$(123) = " 123" and not "123" as might be expected). If the result is negative, instead of a space you get a '-' (minus sign), i.e. STR$(-123) = "-123" and not " -123" as might be expected from the positive behaviour.
2) When converting a float (mumb!, numb#) less than 0.1, the string value may be rendered in 'scientific notation', with 'D' used rather than '*10^' (for example "5.nnnnnnD-02" rather than " .05nnnnnn" or "5.nnnnnn*10^-02"). This only occurs when the number of significant digits needs to be preserved (so .03000000 is rendered as " .03", whilst .030000001 becomes " 3.0000001D-02"), again perhaps not what you might expect.
See also CHR$ for converting an ascii value into a string character.
See also LEFT$, MID$, RIGHT$ for extracting sub-strings from a line of text.
SYSTEM
[edit | edit source]SYSTEM
The .bas exits, the QBasic.exe interpreter is closed and 'control' passes to the Command Window c:\ prompt (or next line of a calling .cmd script etc.)
NOTE!: This only works when you start your program at the command prompt using the "/run" parameter! (EX: "Qbasic /run MyProg.bas") Otherwise, Qbasic assumes you opened your program to make changes, and thus "SYSTEM" drops you back at the editor screen.
THEN
[edit | edit source][Command] [variable] = [value] THEN GOTO [line command value]
Used in conjunction with the GOTO or IF condition commands. It tells the computer what to do if a certain condition has been met.
TO
[edit | edit source][Command] [Variable] = [Value] TO [Value]
Usually used to input a number of variables.
FOR a = 400 TO 500 PRINT a NEXT a
This example will print all numbers from 400 to 500. Instead of declaring all values separately, we can get them all declared in one go.
USING
[edit | edit source]USING "format";
Used to format the output of data from PRINT commands. Normally, the QBasic interpreter will print a number as 8 characters with as many leading spaces as necessary. To change this behavour, the USING command can be used to format the output. For example ..
IF n > 99 THEN PRINT #1, USING "###"; n; ELSE IF n > 9 AND n<=99
THEN PRINT #1, USING "0##"; n; ELSE PRINT #1, USING "00#"; n;
.. will output n from 0 to 999 with leading zeros. Note the ';' after the n. This means 'don't start a new line' and results in the next PRINT #1 adding data directly after the comma (',') Qbasic automatically inserts instead of a line.
VAL()
[edit | edit source]name=VAL([variable$])
Converts the [variable string] contents into a numeric value so it can be used in calculations. If (name) is an INTEGER type, the VAL is rounded down. See also STR$.
A$ = "2"
B$ = "3"
X = VAL(A$) + VAL(B$)
PRINT A$; " + "; B$; " ="; X
WHILE ... WEND
[edit | edit source]WHILE {NOT} [test condition is true] [program code to execute] WEND
The condition is tested and if true (or NOT true) the [program] code is executed until WEND is reached, at which point control passes back to the WHILE line.
WHILE NOT (EOF(1)) LINE INPUT #1, A$ PRINT #2, A$ WEND
While the end of file #1 has not been reached, read each complete line and write it to file #2.
Unlike FOR and DO, it is not possible to EXIT from a WHILE loop
ASCII Chart
[edit | edit source]
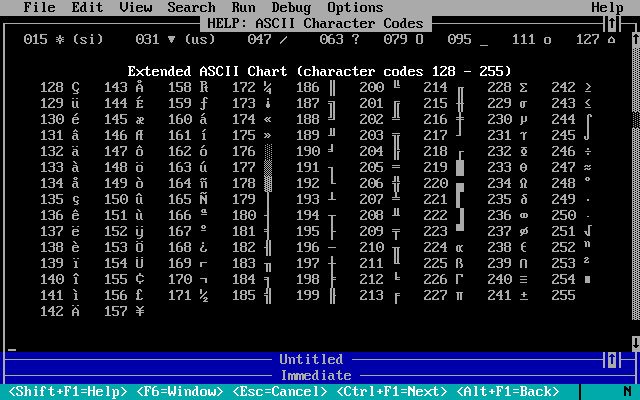