Programming Gambas from Zip/Grammar
Programming[edit | edit source]
Programming is making applications. It is also called ‘coding’ because the instructions are written in ‘code’.
There are many programming languages. Ours is GAMBAS. (Gambas Almost Means BASic). BASIC (Beginner's All-purpose Symbolic Instruction Code) first appeared on 1 May 1964 (55 years ago in 2019, as I write). Everyone loved BASIC. It made programming possible for us ordinary mugs. Benoît Minisini designed and wrote GAMBAS and gives it away free. He lives in Paris, France and is 47 (in 2019). He said, “Programming is one of my passions since I was twelve” and “I am using many other languages, but I never forgot that I have learned and done a lot with BASIC.”

This is a piece of code:
Public Sub Form_Open()
gridview1.Rows.count = 5
gridview1.Columns.count = 1
For i As Integer = 0 To 4
gridview1[i, 0].text = Choose(i + 1, "Red", "Yellow", "Green", "Blue", "Gold")
Next
gridview1.Columns[0].text = "Colour"
End
The language is Gambas. This is a Sub (also called Method, Procedure or Subroutine. If it gave you some sort of answer or result it could be called a Function).

On the left is the design. It is a form. Think of it as a Window. On the right is the application in action. That is what you see when you run the program.
Starting an application is called running it. The program runs. Every statement in the program is executed. The Form_open sub (above) fills the gridview object.
Part 1[edit | edit source]
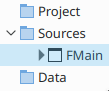
- Open Gambas.
- Double-click FMain in the list on the left. The form opens.
- Look for a GridView among the objects at the bottom right. Drag it onto the form. Adjust its size by dragging the handles (small squares on its edge). Adjust the form size too.
- Right-click the form (NOT the gridview) > Event menu item > Open.
- Type or paste in the code for the open event (as above).
- Press F5 to run your program.
Part 2[edit | edit source]
With the same program that contains the gridview that you just tried, drag a Label onto the form.
Right-click the gridview > Event > Enter, and type this code:
Public Sub gridview1_Enter()
Label1.text = "Hello"
End
Right-click the gridview > Event > Leave, and type this code:
Public Sub gridview1_Leave()
Label1.text = ""
End
Run the program with F5. Move the mouse in and out of the gridview.
Label1.text = "Hello" is a way of saying “Put the word Hello into the text property of Label1.”
Break Complex Things into Parts[edit | edit source]
This is the most important idea about programming. The only way to write a good program is to “divide and conquer”. Start with the Big Idea, then divide it into parts. Piece by piece, write the program. This is called top-down development. The opposite is useful, too: bottom-up development. Write a simple program and keep adding bits and pieces to it until it does all the things you want it to.
The first programs were simple creatures that did but one thing. Then came menus, where you could select one of several things by pressing a key. For example, “Press 1 to get your account balance. Press 2 to recharge. Press 0 to speak to an operator.” Nowadays applications do many things. To choose, you have a menu bar at the top, menus that drop down, and menu items that you click on in each menu. You can click a button. Buttons can be solitary, or friendly with each other and gather together in a toolbar. You can pop up a menu anywhere. You can type shortcut keys to get things to happen. There can be several windows. Within windows (forms) there can be many pages with or without an index tab at the top of each.
Buttons can be on the screen, or the physical keys on your keyboard. The standard keyboard has 101 keys, but they can all be given a second function if you hold down CTRL-, the control key, while you type them. That gives you 202. Not enough? Holding down SHIFT-, the shift key, makes all the keys different again, giving you 303 buttons. There is ALT- (alternative) that makes all the buttons different yet again: 404 of them. The modifier keys held down in combination give you more sets of keys, like more keyboards: SHIFT+CTRL, CTRL+ALT and SHIFT-CTRL-ALT. Now I have lost count. Fortunately for us, no application uses them all.
The key to good programming is to get things into order. Be neat and tidy. Arrange things. Things you do all the time should have their buttons visible; things you do not so often could be hidden away in a popup menu.
Reduce complicated tasks to a series of simple steps. Write lots of little subs, not just one big sub. Write “master subs” that call on lesser subs to do little jobs. The biggest master of them all is the user. You use the program to do this, then that, then something else. The program says, “At your service!” and calls on different subs you have written to do what you want. In the meantime, it waits and listens for your command, checking the keyboard and the mouse, or, if it is not waiting for you, it keeps busy doing something you set it to work on.
Objects, Subs and Events[edit | edit source]
The program above has two things (called Objects): a form, and a gridview.
Things that happen are called Events.
There is an event that “fires” or “is triggered” when a form opens. It is called (no surprise) the OPEN event. If you want something to happen when the form opens, write a sub called Form_Open. The instructions there will be carried out when the OPEN event fires. The form opens when the application starts up.
You have to think of two things: what will happen and when it will happen. The “what” is the list of instructions in Subs. The “when” is a case of choosing which events to handle.
Names and Putting Something into Something[edit | edit source]
Everything has to have a name. People have names. Forms have names. Buttons have names. You get to choose, using letters and numbers, always starting with a letter, and not having any spaces.
The main form is called FMain. It's open event is Form_Open.
I like the convention of calling it FMain. The “F” says, “Form”. If I had a form that listed people's addresses, I would call it FAddress. When it opens—perhaps at the click of a button—its open event would look for a sub called FAddress_Open.
Right-click an object, choose EVENT, and click on the event you want to write some programming for:
- Right-click the form > Event > Open > write code for the Open event.
There will be a big confusing list of events. Don't be fazed: only a few are used often. A big part of learning the language is getting used to the events that are most useful to certain objects. Buttons, for example, like to be Clicked; you don't use the other events very often. Possibly, entering a button might put some help text in a line at the bottom of the window, and leaving it will clear the message, but not often. I have not seen a double-click handler for a button yet: who double-clicks buttons? You will get used to the favourite events that objects have.
- In the program you have written, type or paste this sub. Every time you roll the mouse into the gridview, a message saying “Hello” appears. When you have tried it and it has annoyed you enough, delete the three lines of code.
Public Sub Gridview1_Enter()
Message("You have entered the gridview ... evil laugh...")
End
The Activate event happens when you double-click. Enter these lines and double-click any of the lines.
Public Sub gridview1_Activate()
Message("You double-clicked row " & gridview1.row)
End
gridview1.row is the row you double-clicked on. It is set when you click or double-click. The “&” sign (ampersand) joins two strings of text. (Strings are text ... characters one after the other.) This next code will do something when you click on gridview1:
Public Sub gridview1_Click()
Label1.text = "You clicked on row " & gridview1.Row & ", " & gridview1[gridview1.row, 0].text
End
There are two strings. (1) "You clicked on row " and (2) ", " . Strings have double quotes around them.
What is on the right of the equals goes into what is on the left.
You don't put something into Label1; you put it into the text of Label1.
Usually languages won't allow this: Label1.text = 345. It must be Label1.text = "345" or Label1.text=Str(345), but Gambas doesn't mind. Gridview1.Row refers to the row number you clicked on. Numbers normally go into things that store numbers, and strings go into things that store strings, but Gambas converts it automatically.
gridview1[gridview1.row, 0].text looks complicated, but let's break it down. From the left, it is something to do with gridview1. From the right, it is the text in something. The part in square brackets is two numbers with a comma in between: the row you clicked on and zero. In the example above, it is [2,0]. Row two, column zero.
Gridview rows and Gridview columns start their numbering at zero.
Properties and Kinds of Things[edit | edit source]
In this part the examples are not Gambas, but they are written in Gambas style.
Behold John and Joan:
Parts are referred to like John.arm, Joan.head
Sometimes parts have smaller parts, such as John.hand.finger
Parts have properties, like Joan.height
Some properties are integers (whole numbers), like Joan.Toes.Count
Some properties are floats (numbers with decimal fractions) like John.height . This is not the same as John.Leg.Height .
Some properties are booleans (true/false, yes/no) like Hat On or Hat Off. We could say Persons, as a class, have a property called HatOn, “John.HatOn” or “Joan.HatOn”. (It is true or false.)
They belong to the class called “person”. John is a person; Joan is a person. Persons all have a height, weight, hat-on/hat-off, and other properties.
Persons also have various abilities. The “person” class can sing and wave and smile. Their legs can walk. For example, John.sing(“Baby Face”) . Sometimes John will need to know which version, for example, John.sing(“Baby Face”, “Louis Armstrong”) or Joan.wave(“Hello”, “John”, 8). This means “Joan! Give a hello wave to John, vigorously!”
There will also be events they can respond to, like a push, or when they hear someone singing. These events are John_push() and Joan_push(), John_HearsSinging() and Joan_HearsSinging()
Let's write a response to these events in the style of Gambas.
John might get annoyed when the Push event takes place:
Public Sub John_push()
John.Turn(180)
Message.shout(“Hey! Quit shoving!”)
End
Joan might join in the song that she hears and start singing it too:
Public Sub Joan_HearsSinging(“Ave Maria”)
Joan.HatOn = False
Joan.sing(“Ave Maria”)
End
Boolean properties are yes/no, true/false, haton/hatoff things. Putting false into the HatOn property means taking her hat off before she sings.
John has the ability to turn around, but we must say how much to turn. He turns 180°, doing an about-face so he is facing the person who pushed him.
Mary can sing, but we must say which song to sing. It is the same one that she is hearing. We say that the HearsSinging event is passed the name of the song, “Ave Maria”. That song title is passed to Joan's singing ability, which is referred to as Joan.sing . The bits of information you pass on so that the action can be done are called parameters. Some methods require more than one parameter, and they can be numbers (integers, floats...) or strings (text like “Jingle Bells” or “Fred” or “Quit pushing, will you?!”) or booleans (HatOn/HatOff, Complain/Don’tComplain True/False kinds of things), or other objects or, well, anything.
Let's define a sub and this time we'll put some repetition in it. There are two kinds of repetition—one where you repeat a definite number of times, and another where you have to check something to see if it is time to stop. (And the latter comes in two kinds, where you check if it is time to stop before you start or after you have done it—pretested loops or post-tested loops.) Here are the definite and indefinite types:
Five times:
Public Sub DoExercises()
For i as integer = 1 to 5
Hop()
Next
End
Until tired:
Public Sub DoExercises()
Do Until Tired()
Hop()
Loop
End
While not tired:
Public Sub DoExercises()
While Not Tired()
Hop()
Wend
End
In the first, there will be 5 hops and that is all. “i” is an integer that is created in the line For i as integer = 1 to 5 and it counts from 1 to 5. It is made one bigger by the word NEXT. The repeated section is called a loop.
Tired() is something that has to be worked out. The procedure for working it out will be in another sub somewhere. At the end of it there will be an answer: are you tired or not? Yes or no, true or false. That will be returned as a final value. Tired() looks like a single thing, the value returned by a function. Functions work something out and give you an answer.
“Hop” might need further explanation if “hop” is not already known. You can use any made-up words you like, provided you explain them in terms that are built-in and already known to Gambas. Here is a sub that explains the Hop procedure:
Public Sub Hop()
GoUp()
GoDown()
End
The beauty of breaking procedures down into simpler procedures is that the program explains itself. You are giving names to the complicated tasks without getting lost in the fine detail of how they are done. Not only can you use words that make sense to someone else reading your program but you can track down errors more easily. You can test each part more easily. You can also modify your program more easily. DoExercises() can be left as it is, but you can change the definition of hopping with
Public Sub Hop()
GoUp()
ShoutAndPunchTheAir()
GoDown()
End
or
Public Sub Hop()
GoUp(LeftFoot)
GoDown()
GoUp(RightFoot)
GoDown()
End
Comments[edit | edit source]
Anything after a single apostrophe is a note to yourself. Gambas disregards it. Put in comments to remind yourself of what you are doing. It can also explain your thinking to someone else who might need to read your program. Here is some more pseudocode:
Public Sub Joan_HearsSinging(“Ave Maria”) 'you must say what the song is
Joan.HatOn = False 'remove your hat
'now the fun starts
Joan.sing(“Ave Maria”) 'sing the same song
End
That ends the pseudocode. The next section is back into Gambas.