OpenGL Programming/Basics/Color
Color[edit | edit source]
Using monitors RGB to define a single color[edit | edit source]
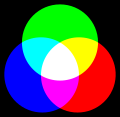
There are many ways to specify a color in computer graphics, but one of the simplest and most widely used methods of describing a color is the RGB color model. RGB stands for the colors red, green and blue: the additive primary colors. Each of these colors is given a value, in OpenGL usually a value between 0 and 1. 1 means as much of that color as possible, and 0 means none of that color. We can mix these three colors together to give us a complete range of colors, as shown to on the left.
For instance, pure red is represented as (1, 0, 0) and full blue is (0, 0, 1). White is the combination of all three, denoted (1, 1, 1), while black is the absence of all three, (0, 0, 0). Yellow is the combination of red and green, as in (1, 1, 0). Orange is yellow with slightly less green, represented as (1, 0.5, 0).
For more information on RGB, see the Wikipedia article.
Using glColor3f[edit | edit source]
glColor3f() takes 3 arguments: the red, green and blue components of the color you want. After you use glColor3f, everything you draw will be in that color. For example, consider this display function:
void display() { glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); glColor3f(0.5f, 0.0f, 1.0f); // (0.5, 0, 1) is half red and full blue, giving dark purple. glBegin(GL_QUADS); glVertex2f(-0.75, 0.75); glVertex2f(-0.75, -0.75); glVertex2f(0.75, -0.75); glVertex2f(0.75, 0.75); glEnd(); glutSwapBuffers(); }
This outputs a rectangle/quad in an attractive purple color.
Play with glColor3f(), passing different arguments in. See if you can make dark green, light gray or teal.
Giving Individual Vertices Different Colors[edit | edit source]

glColor3f can be called in between glBegin and glEnd. When it is used this way, it can be used to give each vertex its own color. The resulting rectangle is then shaded with an attractive color gradient, as shown on the right.
void display() { glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); glBegin(GL_QUADS); glColor3f(0.5f, 0.0f, 1.0f); // make this vertex purple glVertex2f(-0.75, 0.75); glColor3f(1.0f, 0.0f, 0.0f); // make this vertex red glVertex2f(-0.75, -0.75); glColor3f(0.0f, 1.0f, 0.0f); // make this vertex green glVertex2f(0.75, -0.75); glColor3f(1.0f, 1.0f, 0.0f); // make this vertex yellow glVertex2f(0.75, 0.75); glEnd(); glutSwapBuffers(); }