PyGame Guide/Getting Ready
About this guide
[edit | edit source]
What does this guide cover?
First, this guide covers the basic syntax and usage of the Python language in case you are coming from another language. If you've already spent time programming in Python, you can skip the "Python Crash Course" section.
Second, this guide also covers the PyGame basics that you will need in order to build a functioning 2D game. The "PyGame Crash Course" runs through all the basic functionality pretty quickly, complete with example code.
After we get the basics of Python and PyGame down, we will dive into building a few simple 2D games in "The PyGame Cook Book".
Finally, there is a "Quick Reference" guide for both Python and PyGame, followed by a Glossary in case you need a refresher on any terms used in this book.
Prerequisites
[edit | edit source]What prior experience do I need? This guide works best if you have already had some prior programming experience in an object oriented language, such as C++, C#, and/or Java. In the "Python Crash Course" chapter, I go over some of the basics of Python so that you can be familiar with its syntax, but it is not an introductory programming course and it doesn't cover theory and the basics you would have had in a previous course.
What if I haven't programmed before?
You might be able to get started with 2D programming using just this guide if you haven't had any programming classes before, but you will hit a wall on the difficulty of problems that you can solve -- but don't worry, there are plenty of free guides online that you can use to study up!
What is Python and PyGame?
[edit | edit source]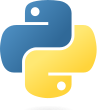
Python is an interpreted high-level programming language. In this case, "high level" means further from machine code. "High level" doesn't mean it is harder - actually, high-level languages are generally easier to use than low-level languages like C. As a language, Python can be written in an Object-Oriented Style (which should be familiar if you're from the C++/C#/Java side of things), but it also supports other programming paradigms - or other ways of structuring and writing code. I prefer writing games using Object Oriented techniques.
PyGame is a library that can be used with Python. A library is pre-written code that can be reused in multiple programs. This usually includes functions and classes that provide new functionality.
PyGame handles features like:
- Loading and drawing graphics
- Loading and playing sounds
- Loading fonts and drawing text
- Detecting keyboard and mouse input
Additionally, PyGame is built to be cross-platform, so you can write a game on your machine, and your friends can run the game on Linux, Mac, Windows, or even mobile devices!
Setting up Python and PyGame
[edit | edit source]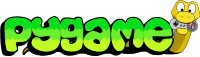
Downloading PyGame
[edit | edit source]The PyGame website has the downloads for PyGame, as well as a tutorial on getting started, documentation for PyGame, and even a directory of games made with PyGame. Once you create a game, you can put your game on the directory, too! The PyGame website is at pygame.org
Windows
[edit | edit source]For Windows, you will download a version of PyGame from here: http://pygame.org/download.shtml
Look for the "Windows" header and download the version of PyGame that contains "py2.7" in the title. At the time of writing, the latest version is pygame-1.9.1.win32-py2.7.msi.
Python Versions
Version 2 and Version 3 of Python have a lot of differences and are not always compatible between each other. This version of PyGame uses Python 2.7. |
(Windows instructions work in progress)
Linux
[edit | edit source](Linux instructions work in progress)

If you're using Ubuntu, Linux Mint, or Debian, you should be able to install Python via the Synaptic Package Manager, or even the Software Manager.
Search for "pygame", and you should download the python-pygame package. It should also install the Python dependencies at the same time.
Mac
[edit | edit source](Mac instructions work in progress)
For Mac, you will download a version of PyGame from here: http://pygame.org/download.shtml
Look for the "Macintosh" header and download the version for py2.7.
Downloading a text editor
[edit | edit source]Source code is all text, so you will need a decent text editor to write with. The Windows default Notepad is a terrible program, so you should download something else.
Geany
[edit | edit source]Geany is a free, cross-platform editor. You can also install additional plugins into Geany to customize it. Download it at: http://www.geany.org/Download/Releases
(Text editor instructions work in progress)
Testing it out
[edit | edit source]Let's make sure that Python and PyGame are working correctly with some small example programs. You can re-type the code given in this document, or you can download the files from the guide repository at bitbucket.org/moosaderllc/rachels-pygame-guide
Making sure Python works
[edit | edit source]First, create a directory on your computer for your Python projects, and create a folder for this project (\Testing Python" or something). First we will build just a Python program, and then we will build a PyGame program.
In Geany, create a new file, and save it to your project directory as simple python.py. Make sure it has the .py extension - all Python source files must end with this.
Windows note!
If you're in Windows, your folder options might be set so that file extensions are hidden by default. You might want to set all extensions to be visible so that you don't get confused! |
Add the following code into your source file:
print( "Hello, world!" )
for i in range( 1, 10 ):
print( i )
If you're using Geany, you can run the Python program by hitting F5.
Example output
[edit | edit source]Once it has run, the Python program output should look like this:
Hello, world! 1 2 3 4 5 6 7 8 9
Making sure PyGame works
[edit | edit source]Next we will create a simple PyGame test to make sure we can get a window open. Create another source file named simple pygame.py. Paste in the following code. Don't worry if you don't understand the code yet - this is just to make sure everything was set up properly!
import pygame, sys
from pygame.locals import *
pygame.init()
timer = pygame.time.Clock()
window = pygame.display.set_mode( ( 300, 300 ) )
pygame.display.set_caption( "Testing out PyGame!" )
# Game loop
while True:
window.fill( pygame.Color( 50, 200, 255 ) )
# Check input
for event in pygame.event.get():
if ( event.type == QUIT ):
pygame.quit()
sys.exit()
# Update screen
pygame.display.update()
timer.tick( 30 )
Once you run it, you should get a small window with a light blue background.

You can close the window once you're done.
What if it's not working?!
Sometimes this happens! If you're having trouble running the program, there are a few things you can do to debug it...
- Double-check all text and symbols for typos - these kinds of errors are easy to make! Also make sure your capitalization is regular, PyThOn Is CaSe SeNsItIvE!
- Download the files from repository and try running that instead. Compare my code and your code to see what differs.
- Do a search for the error message that you're getting - chances are that somebody else has had that same problem!
How do I download the files from the repository?
You can find the repository at this link: https://bitbucket.org/moosaderllc/rachels-pygame-guide/. Once there, you can use the Clone button if you're familiar with Git, or you can click on Downloads to find a link to download all the files to your computer.
What's next?
Good work! Next we will run through some of the features of Python so you can become familiar with its syntax!