OpenGL Programming/Installation/GLUT
Jump to navigation
Jump to search
GLUT is the utility toolkit that provides Window management for OpenGL applications and their interface with the operating system.
GLUT alternatives
[edit | edit source]GLUT started as a non-free library. Nowadays, free/open-source and portable alternatives such as FreeGLUT and OpenGLUT exist.
In this wikibook, we recommend FreeGLUT. See the platform-specific installation pages for details on how you can install it.
Using GLUT to discover OpenGL version
[edit | edit source]#include <stdio.h> /* printf */
#include <GL/glut.h> /* glut graphics library */
/*
Linux c console program
gcc f.c -lglut -lGL
./a.out
*/
int main(int argc, char **argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutCreateWindow("red 3D lighted cube");
printf("GL_VERSION = %s\n",glGetString(GL_VERSION) ); /* GL_VERSION = 2.1.2 NVIDIA 195.36.24 */
return 0;
}
More information
[edit | edit source]This program shows info about glut.[1]
To compile :
gcc v.c -lglut -lGL
To run :
./a.out
#include <stdio.h>
#if defined(HAVE_FREEGLUT)
#ifdef WIN32
#include "../include/GL/freeglut.h"
#else
#include <GL/freeglut.h>
#endif
#else
#include <GL/glut.h>
#endif
#define PROGRAM "glversion"
int main(int argc, char **argv)
{
char *version = NULL;
char *vendor = NULL;
char *renderer = NULL;
char *extensions = NULL;
GLuint idWindow = 0;
int glutVersion;
glutInit(&argc, argv);
glutInitWindowSize(1,1);
glutInitDisplayMode(GLUT_RGBA);
idWindow = glutCreateWindow(PROGRAM);
glutHideWindow();
glutVersion = glutGet(0x01FC);
version = (char*)glGetString(GL_VERSION);
vendor = (char*)glGetString(GL_VENDOR);
renderer = (char*)glGetString(GL_RENDERER);
extensions = (char*)glGetString(GL_EXTENSIONS);
printf("GLUT=%d\nVERSION=%s\nVENDOR=%s\nRENDERER=%s\nEXTENSIONS=%s\n",
glutVersion,version,vendor,renderer,extensions);
glutDestroyWindow(idWindow);
return(0);
}
< OpenGL Programming/Installation
Browse & download complete code 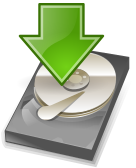
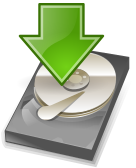